Requirements
Latest version of the WooCommerce API Manager.
WC API Manager PHP Library version 2.9.x
This library can be dropped into a WordPress plugin or theme to activate/deactivate API Keys, to check for Software updates, and check an API Key’s status with the WooCommerce API Manager. If the client server uses WordPress 5.5, then auto-updates are available.
The wc-am-client.php
file should be placed in the plugin’s root folder, or the same level as the functions.php
file if it is a theme. The code below goes in the root plugin file, or a theme’s functions.php file, and should always be the first code in the file.
The Class Name WC_AM_Client
can be changed to something unique if desired.
The variable $wcam_lib
from the example is optional and is not required, but must have a unique name if used to check if the API Key has been activated before allowing use of the plugin/theme.
In the example code, if the $product_id value is an empty string the customer can manually enter the product_id into a form field on the activation screen as shown in this screenshot below.
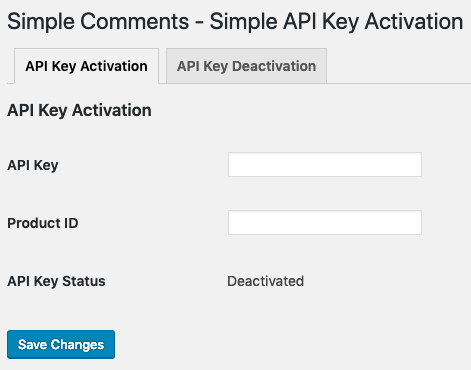
IMPORTANT: The code block below should be added just below your plugin header, or the top of your functions file in a theme. The code block below should always be loaded first, so it will always work, and won’t break in case something else in your plugin or theme breaks.
Example Code to add to Plugins and Themes
// Exit if accessed directly
defined( 'ABSPATH' ) || exit;
/**
* IMPORTANT
*
* Use only the code below at the top of a plugin file below the plugin header, or at the top of a theme functions file.
*/
// Load WC_AM_Client class if it exists.
if ( ! class_exists( 'WC_AM_Client_2_9' ) ) {
/*
* |---------------------------------------------------------------------
* | This must be exactly the same for both plugins and themes.
* |---------------------------------------------------------------------
*/
require_once( plugin_dir_path( __FILE__ ) . 'wc-am-client.php' );
}
// Instantiate WC_AM_Client class object if the WC_AM_Client class is loaded.
if ( class_exists( 'WC_AM_Client_2_9' ) ) {
/**
* This file is only an example that includes a plugin header, and this code used to instantiate the client object. The variable $wcam_lib
* can be used to access the public properties from the WC_AM_Client class, but $wcam_lib must have a unique name. To find data saved by
* the WC_AM_Client in the options table, search for wc_am_client_{product_id}, so in this example it would be wc_am_client_132967.
*
* All data here is sent to the WooCommerce API Manager API, except for the $software_title, which is used as a title, and menu label, for
* the API Key activation form the client will see.
*
* ****
* NOTE
* ****
* If $product_id is empty, the customer can manually enter the product_id into a form field on the activation screen.
*
* @param string $file Must be __FILE__ from the root plugin file, or theme functions, file locations.
* @param int $product_id (Optional, can be an empty string) Must match the Product ID number (integer) in the product if set.
* @param string $software_version This product's current software version.
* @param string $plugin_or_theme 'plugin' or 'theme'
* @param string $api_url The URL to the site that is running the API Manager. Example: https://www.toddlahman.com/ Must be the root URL.
* @param string $software_title The name, or title, of the product. The title is not sent to the API Manager APIs, but is used for menu titles.
*
* Example:
*
* $wcam_lib = new WC_AM_Client_2_9( $file, $product_id, $software_version, $plugin_or_theme, $api_url, $software_title );
*/
// Default Menu Theme example.
//$wcam_lib = new WC_AM_Client_2_9( __FILE__, 234, '1.0', 'theme', 'http://wc/', 'WooCommerce API Manager PHP Library for Plugins and Themes' );
/*
* Default Menu Plugin example.
*
* Second argument must be the Product ID number if used. If left empty the client will need to enter it in the activation form.
* The $wcam_lib is optional, and must have a unique name if used to check if the API Key has been activated before allowing use of the plugin/theme.
* Remember if the $wcam_lib variable is set it will be globally visible.
*
* In the two examples below, one has the Product ID set, and the other does not, so it will be an empter string. If the Product ID is not set
* the customer will see a form field when activating the API Key that requires the Product ID along with a form field for the API Key.
* Setting the Product ID below will eliminate the required form field for the customer to enter the Product ID, so the customer will only
* be required to enter the API Key. If you will offer the product as a variable product where the customer can switch to another product
* with a different Product ID, then do not set the Product ID here.
*/
//$wcam_lib = new WC_AM_Client_2_9( __FILE__, 32960, '1.2', 'plugin', 'http://wc/', 'WooCommerce API Manager PHP Library for Plugins and Themes' );
$wcam_lib = new WC_AM_Client_2_9( __FILE__, '', '1.2', 'plugin', 'http://wc/', 'WooCommerce API Manager PHP Library for Plugins and Themes' );
}
Custom Top Level or Top Level Submenu Code
/**
* Custom top level or top level submenu.
*
* Last argument to the WC_AM_Client_2_9 class is to prevent the not activated yet admin message from being displayed, which may not be necessary with a custom menu.
*
* Example using add_submenu_page( $parent_slug, $page_title, $menu_title, $capability, $menu_slug, $callback = '', $position = null );
*
* Argument must be passed as an array:
*
* $file, $product_id, $software_version, $plugin_or_theme, $api_url, $software_title = '', $text_domain = '', $custom_menu = array(), $inactive_notice = true
* Only arguments with values need to be provided.
*
* Custom menus allowed:
*
* add_submenu_page( $parent_slug, $page_title, $menu_title, $capability, $menu_slug, $callback = '', $position = null );
* add_options_page( $page_title, $menu_title, $capability, $menu_slug, $callback = '', $position = null );
* add_menu_page( $page_title, $menu_title, $capability, $menu_slug, $callback = '', $icon_url = '', $position = null );
*
*/
// $wcam_lib_custom_menu = array( 'menu_type' => 'add_submenu_page', 'parent_slug' => 'my-plugin.php', 'page_title' => 'My Plugin License Activation', 'menu_title' => 'API Key' );
// $wcam_lib = new WC_AM_Client_2_9( __FILE__, 168804, '1.2', 'plugin', 'http://wc/', 'WooCommerce API Manager PHP Library for Plugins and Themes', 'wc-am-text', $wcam_lib_custom_menu, false );
How to Activate and Update in a Multisite Environment
The first Subdomain or Subdirectory in the network, aka site1, must have the plugin or theme activated with the API Key for software updates to work. There is only one copy of the plugin or theme, and that copy is always updated from site 1. If the client purchase a single API Key activation, but uses it on a WordPress Multisite installation, the client will need two API Key activations, one for the default site 1, and another for the site number the software will be used on. The plugin or theme cannot be network activated, but must have the API Key activated per site.
Preventing Unauthorized Plugin or Theme Use Before API Key Activation
To prevent a customer from using your software until after activating it with the API Key, you can surround the code that loads your software with the following if condition:
/**
* Above this line should be the code that loads the WC API Manager PHP Library, which must be placed in the root plugin file.
* Do not use the true argument in the function get_api_key_status() if it is used, as shown in this example, to protect software use until after API Key activation.
* When the argument is set to false, which is the default when no argument is used, the result comes from the saved value in options table.
* When argument is set to true, the result comes from the WC API Manager on the live store server.
*/
if ( is_object( $wcam_lib ) && $wcam_lib->get_api_key_status() ) {
// Code to load your plugin or theme here.
// This code will not run until the API Key is activated.
}
Client Testing
For all client testing, especially when attempting resolve client errors, or to make sure client API queries are working as expected, use https://www.postman.com/pricing/. The product can also be used to perform testing, but Postman will return all the API response information that can reveal what is really happening behind the scenes.
The PHP Library includes a postman_api_query_example_collection.json file that can be imported into Postman as a template to begin testing as an API client to connect to your store.
Client Errors
Error – Cannot activate the API Key.
Problem:
Cannot activate the API Key.
Plugin Solutions:
- If the version of the PHP Library is < 2.8:
- Go to Settings > API Key Deactivation tab, check the box for Deactivate API Key, then Save changes. If you do not see a message with the number of API Key activations remaining try the next option.
- Go to Plugins, deactivate then reactivate the plugin. Go to Settings > activation menu for the plugin and reactivate the API Key. If you do not see a message with the number of API Key activations remaining try the next option.
- This last step can also be the first step if preferred. Have the customer go to your WooCommerce store My Account dashboard > API Keys, and delete the activation for the device or web site. The store manager can also delete the activation on the order screen. The https://wordpress.org/plugins/user-switching/ plugin can also be used by the store manager to login as the customer on their My Account dashboard to delete the API Key activation. Now try to activate the API Key on the customer device or web site.
- If the version of the PHP Library is > 2.8:
- Have the customer go to your WooCommerce store My Account dashboard > API Keys, and delete the activation for the device or web site. The store manager can also delete the activation on the order screen. The https://wordpress.org/plugins/user-switching/ plugin can also be used by the store manager to login as the customer on their My Account dashboard to delete the API Key activation.
Themes Solutions:
- If the version of the PHP Library is < 2.8:
- Go to the API Key Deactivation tab, check the box for Deactivate API Key, then Save changes. If you do not see a message with the number of API Key activations remaining try the next option.
- If a second theme is installed switch to another theme, then switch back. If a second theme is not installed, then install the latest WordPress theme to make the temporary switch. Go to Settings > activation menu for the plugin and reactivate the API Key. If you do not see a message with the number of API Key activations remaining try the next option.
- This last step can also be the first step if preferred. Have the customer go to your WooCommerce store My Account dashboard > API Keys, and delete the activation for the device or web site. The store manager can also delete the activation on the order screen. The https://wordpress.org/plugins/user-switching/ plugin can also be used by the store manager to login as the customer on their My Account dashboard to delete the API Key activation. Now try to activate the API Key on the customer device or web site.
- If the version of the PHP Library is > 2.8:
- Have the customer go to your WooCommerce store My Account dashboard > API Keys, and delete the activation for the device or web site. The store manager can also delete the activation on the order screen. The https://wordpress.org/plugins/user-switching/ plugin can also be used by the store manager to login as the customer on their My Account dashboard to delete the API Key activation.
Error – An error occurred while updating … : Update package not available.
Problem:
An error occurred while updating … : Update package not available.
Possible Solutions:
- Activate the API Key if it is not already.
- Go to the product edit screen and click Update to update the product page. This is where the metadata is stored that the API Manager uses. An update tries to refresh the WooCommerce product cache that may be causing the issue.
- Deactivate the API Key, go to Plugins and delete the plugin and reinstall it, then reactivate the API Key.
Error – The API Key has already been activated …
Problem:
Unable to activate API Key due an error such as “Cannot activate API Key. The API Key has already been activated with the same unique instance ID sent with this request.”
Solution:
- Go to the My Account dashboard, and delete the activation for the object, or the client will end up with more than one activation for the same object. The activation error was returned because the client used an instance ID that was incorrect, which is unique, and was for another activation.
- The store manager can also delete an activation on the order screen for the client.
Error – Connection failed to the License Key API server. Try again later.
Problem:
Unable to activate API Key due an error such as “Connection failed to the License Key API server. Try again later.” the problem is most likely one of these possibilities:
- There may be a problem with the client server preventing outgoing requests.
- The store the client is connecting to is blocking the client’s API request.
- Your store may be under attack, such as a DDOS (distributed denial of service).
- If your store uses an SSL/TLS site certificate used for HTTPS connections, it could be expired. This library requires a valid and active certificate to protect clients.
- A SSL/TLS site certificate used for HTTPS connections is HIGHLY RECOMMENDED for store and customer security.
Possible Solutions:
- Have the client disable CloudFlare, or other CDNs, all firewall and caching software.
- The API Manager store should also disable CloudFlare, or other CDNs, all firewall and caching software. Any of these can mangle HTTP/S requests. Also make sure the client software is not trying to connect using HTTP, and being forwarded to HTTPS, as this can cause breakage in some cases. The rule of thumb is to start working with the API Manager without a firewall or CDN, then add these services if desired, but always test to make sure everything is still working.
- In the case of a DDOS, you may need to carefully add CloudFlare, or some other firewall service. This could make things worse if you’re not sure how to implement a firewall, so get help if some things are not working as expected.
- Use https://www.ssllabs.com/ssltest/ to check that your HTTPS connections are working properly.
- If a command line is available, and you can use CURL, use the command “curl -I your-root-site-url”.
API Server Hosting
The API Manager listens for API requests, so it is critical nothing block those requests. Having your own dedicated virtual server eliminates issues with a web hosting environment blocking queries intended for the API without your knowledge, leaving you to troubleshoot issues you can’t see. If you have any issues consider hosting your site at Digital Ocean.
Screenshots

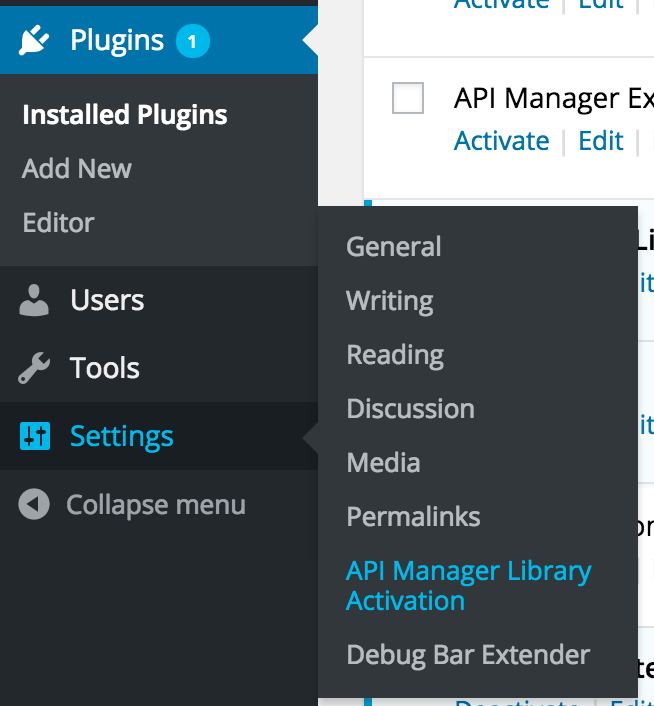
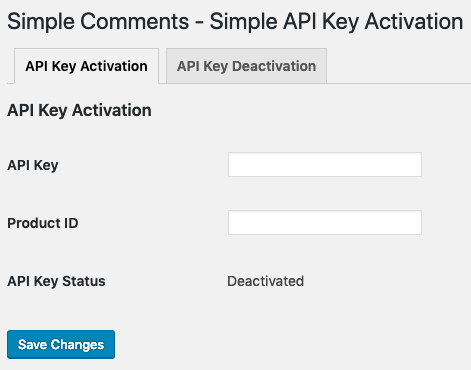
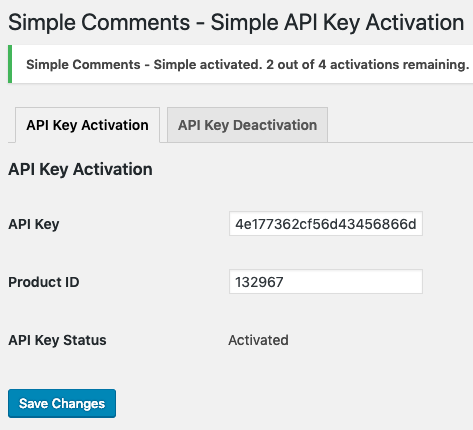
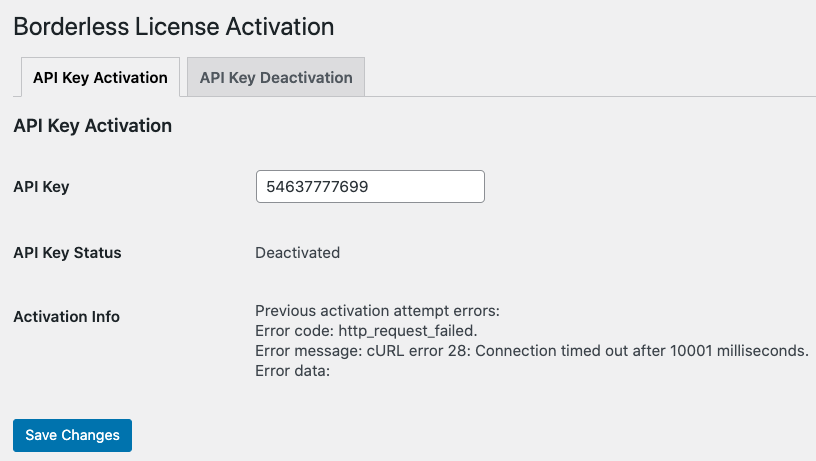
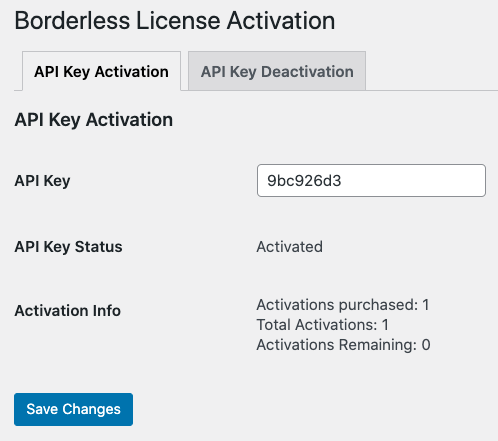
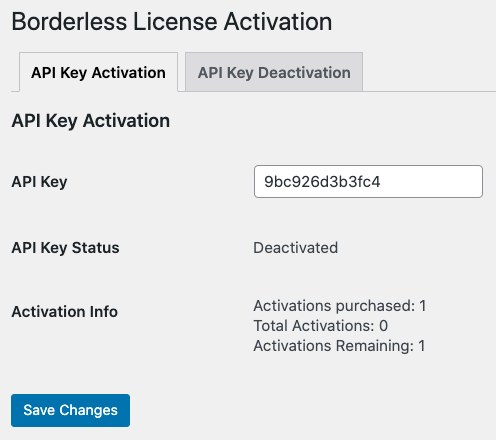
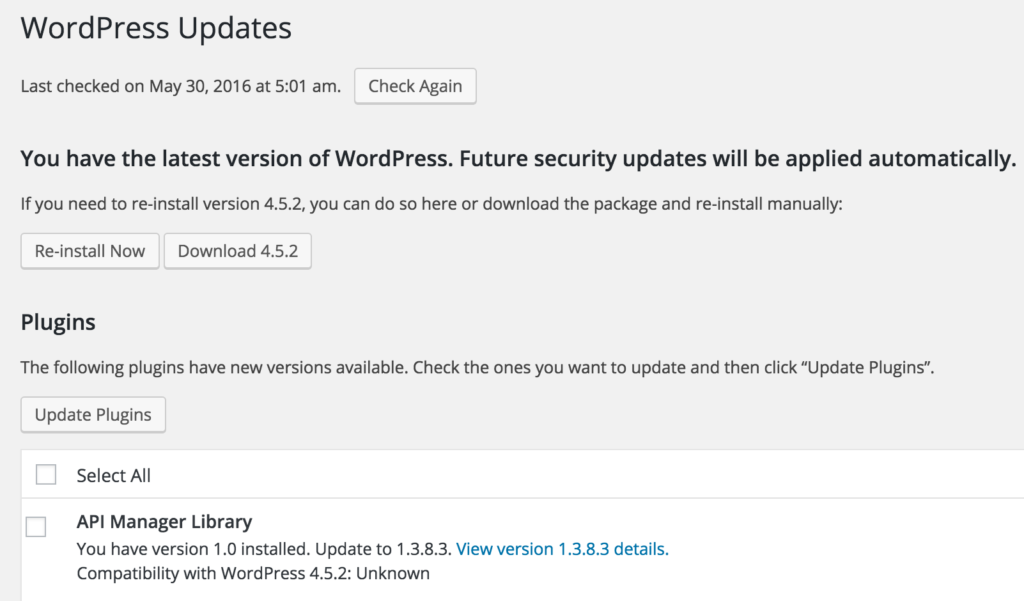

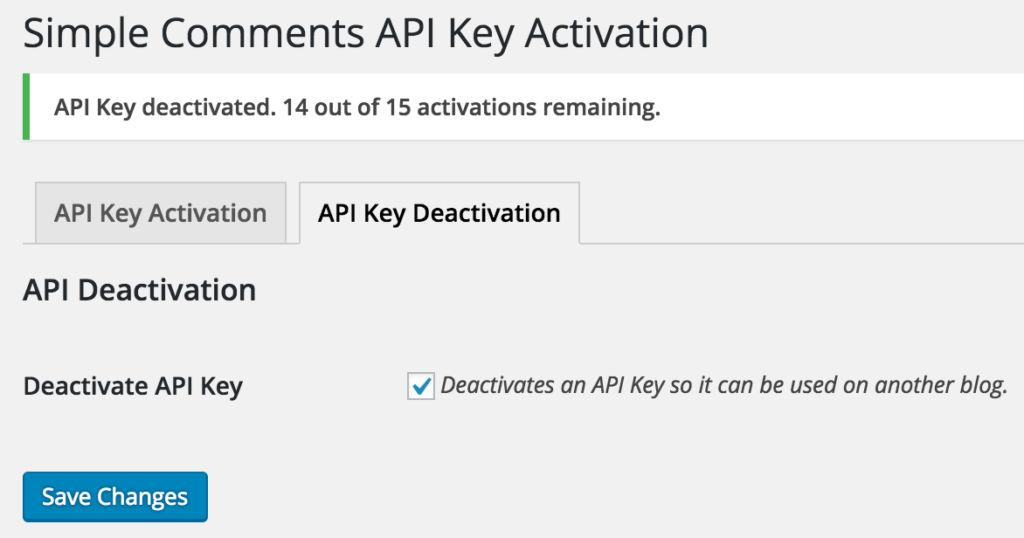
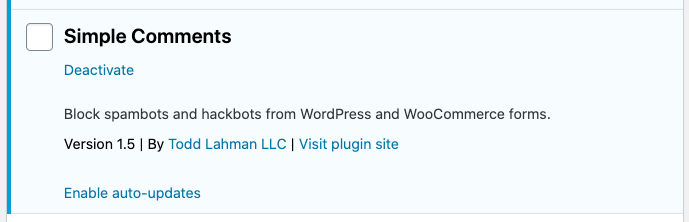
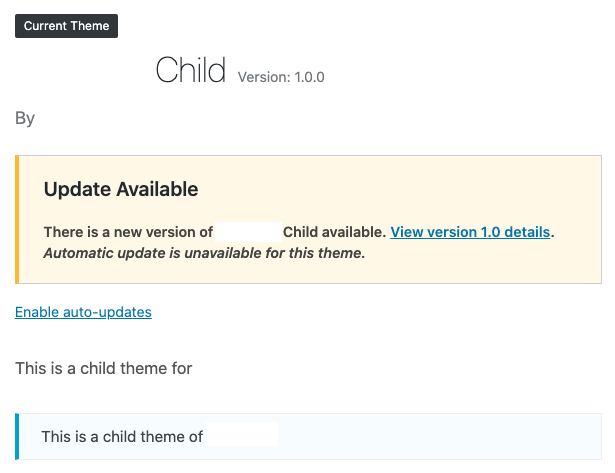